In this post we are going to learn how colors are created on our LED matrix. Let’s start by talking about what an LED is.
Light Emitting Diodes (LEDs)
You’ve probably heard a lot about LED lights because they are the most common way that we light our homes these days. This was not always the case. Up until quite recently, the most common approach to lighting was to use incandescent bulbs. This approach to generating light is pretty easy to understand. When you heat a material up to a very high temperature it glows. For example, iron or glass that is melted glows red hot until it cools to the desired shape. The same is the case for an incandescent bulb where a tiny filament reaches such a high temperature that it glows yellow.
At some point it was discovered that a certain type of electronic component, the diode, produces light in a way that does not require the material to heat up to high temperatures. To understand what a diode is we first need to talk about semiconductors. We all know that if we put a metal object into an outlet we are going to get shocked because the electricity conducts through the metal object and into our bodies. We also know that some materials, like the plastic on an electrical cable, are insulators. If we hold a cable with an electrical charge we do not get shocked because the electricity cannot conduct through the insulator and into our bodies. It turns out that some materials known as semiconductors are somewhere in between conductors and insulators. A semiconductor’s ability to conduct electricity can be changed in a number of ways such as by varying the temperature, light, or pressure in the environment or applying a small electrical charge. For this reason, a semiconductor can act as a controller of electricity which is super useful in building circuits. As an example, if you want to make a circuit where your coffee maker turns on when it detects light, you could use a photosensitive semiconductor to drive that logic. Computers are made from billions of semiconducting elements so you can imagine just how complex their logic can be!
So now let’s talk about diodes. A diode is a semiconductor that only allows electricity to flow in one direction. It’s kind of like a one way street. At some point it was discovered that some diodes glow when electricity is flowing through them. By using different chemicals, scientists were able to create diodes that glow with different colors.
The LED matrix is basically a bunch of LEDs that have been wired together. If you look carefully at the matrix you can see individual LEDs separated by three gold colored stripes. The stripes in between the LEDs transfer power and data through the strip. Each LED actually has three smaller LEDs inside of it with the colors red, green, and blue. These smaller LEDs individually light up and have the letters R, G, and B in the figure below. The circled component is the integrated circuit (IC) which drives the LEDs and is connected to each LED via a tiny gold wire.
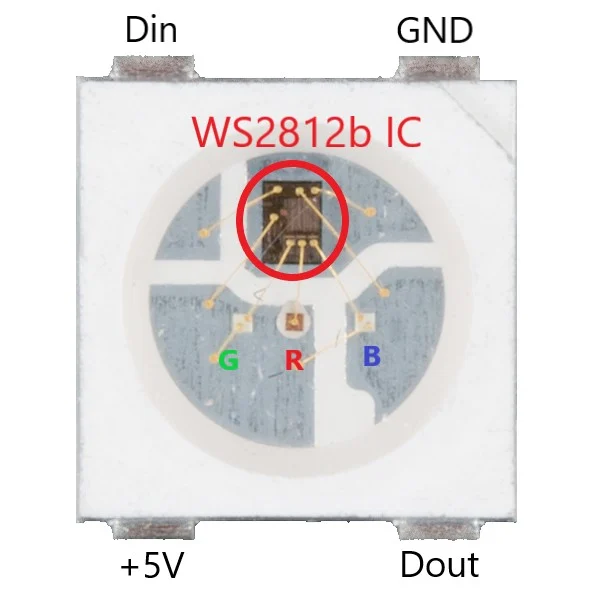
At this point you might be wondering why the LEDs have red, green, and blue mini-LEDs inside of them? It turns out that with those three colors, you can create a large variety of other colors.
Programming an LED
Let’s see how we can program our light board to show different colors. We’ll start with this example that illuminates three pixels with three different colors.
Remember this little bit of code:
red = (255, 0, 0)
What exactly is this code doing? The three numbers inside of the parenthesis correspond to the intensity of red, green and blue that the LED should emit. Each number can range from 0 to 255. The value (255, 0, 0) is setting maximum intensity to red, and zero intensity to green and blue. That’s why the LEDs in the first lesson were shining bright red.
Let’s go ahead and play around with this and see what we can do! Try the code below.
from wc import *
canvas = Canvas()
canvas.add([Point(0,0,'red'), Point(1,0,'green'), Point(2,0,'blue')])
When you save this code you will see red, green and blue appear on the light board as shown below.
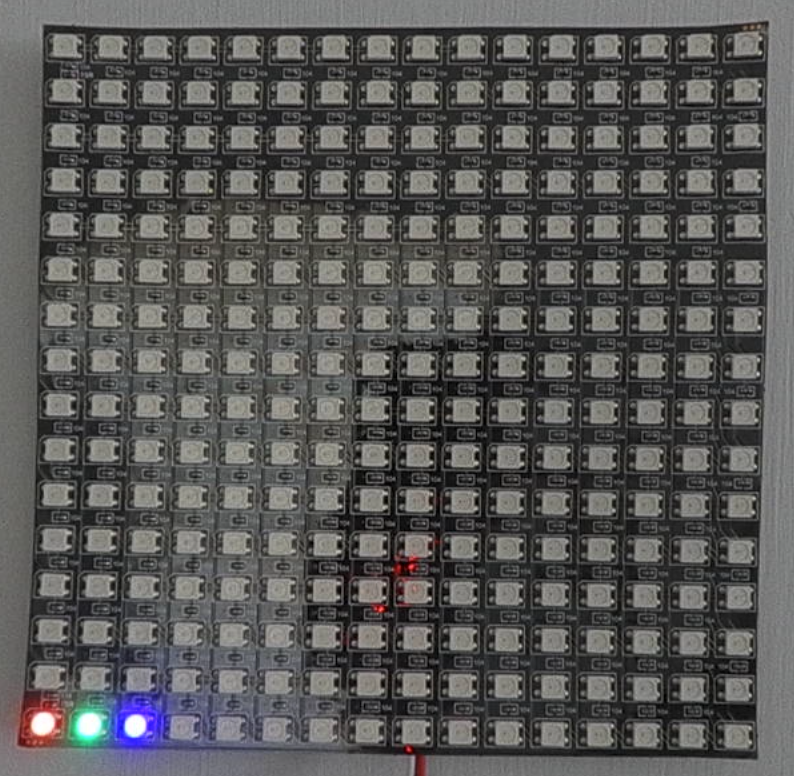
A handful of colors have been predefined in the library including red, blue, green, yellow, purple, cyan, white
and black
. You can however create a variety of other colors using a more complicated notation where the quantities of red, green and blue can be controlled individually. This will be explained in more detail in the next section which discusses the color wheel. For now, let’s try to create some other colors. The code below introduces three colors which have been labeled my_color_1, my_color_2, my_color_3
.
from wc import *
canvas = Canvas()
my_color_1 = (255,100,100)
my_color_2 = (100,255,100)
my_color_3 = (100,100,255)
canvas.add([Point(0,0,my_color_1), Point(1,0,my_color_2), Point(2,0,my_color_3)])
It is also possible to code the above with this abridged syntax.
from wc import *
canvas = Canvas()
canvas.add([Point(0,0,(255,100,100)), Point(1,0,(100,255,100)), Point(2,0,(100,100,255))])
This approach of not using variables reduces your typing but it also makes the code much harder to understand. When we code we should always try to make things as clear as possible so that other coders can understand our code. Using variable names that are intuitive and make sense to others is an important part of that.
The Color Wheel
You might have heard in a science class that combining different colors allows you to create other colors. The diagram below depicts this concept. For example, if you mix red and green you get yellow and if you mix red and blue you get purple.
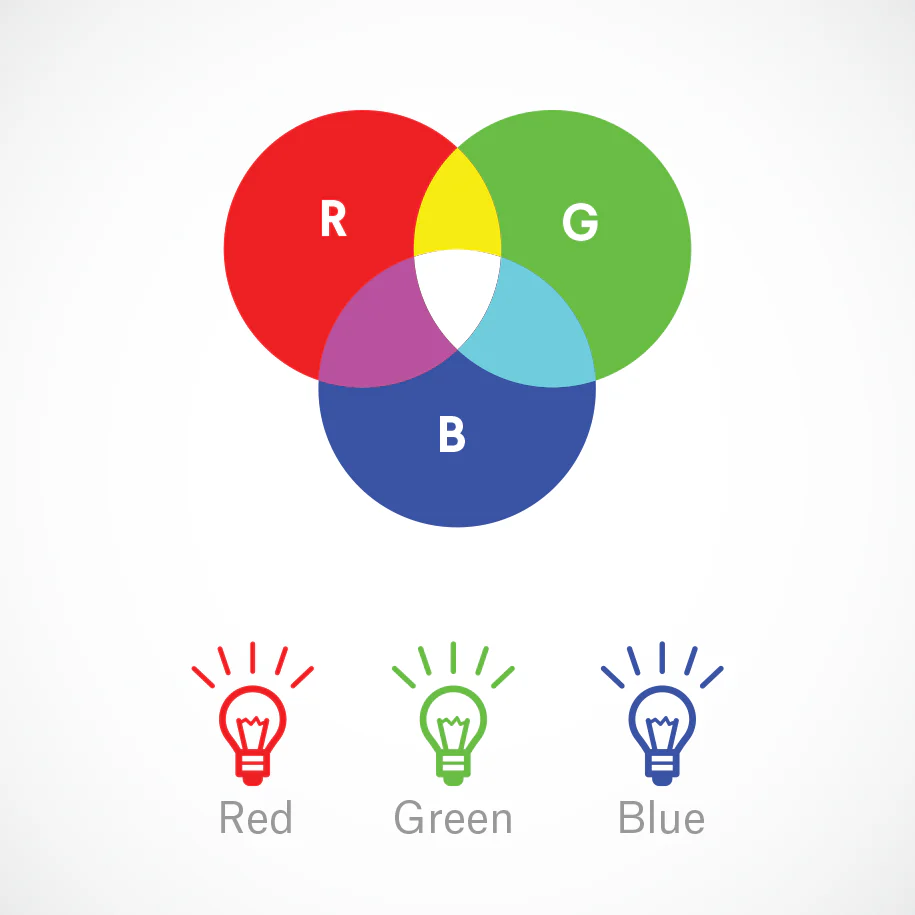
Let’s try to do this and see if it works on our light board.
from wc import *
canvas = Canvas()
yellow = (255,255,0)
purple = (255,0,255)
canvas.add([Point(0,0,yellow), Point(1,0,purple)])
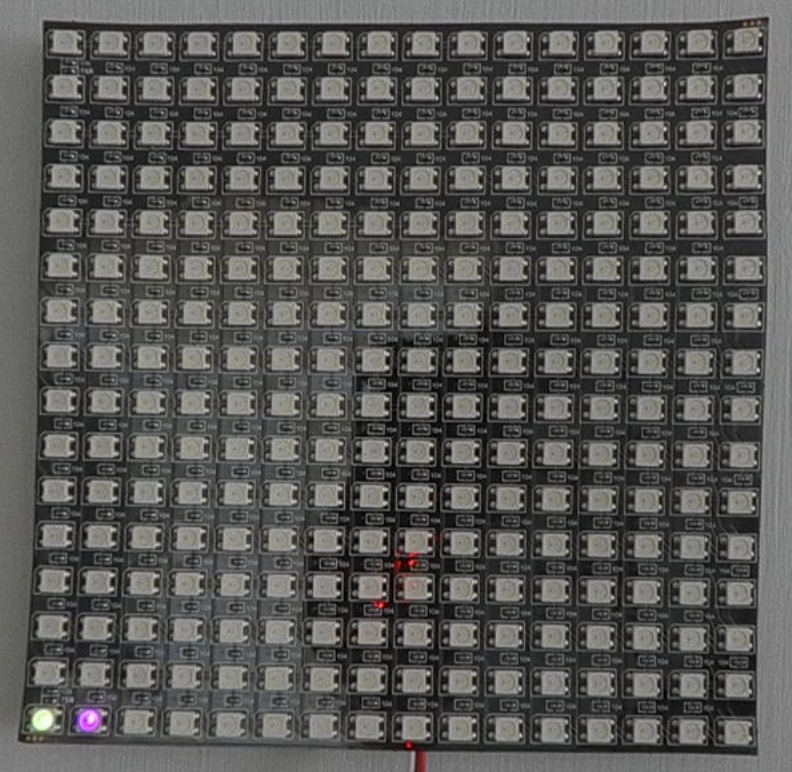
If you mix all three colors together you get white
from wc import *
canvas = Canvas()
white = (255,255,255)
canvas.add(Point(0,0,white))
This results in the following display
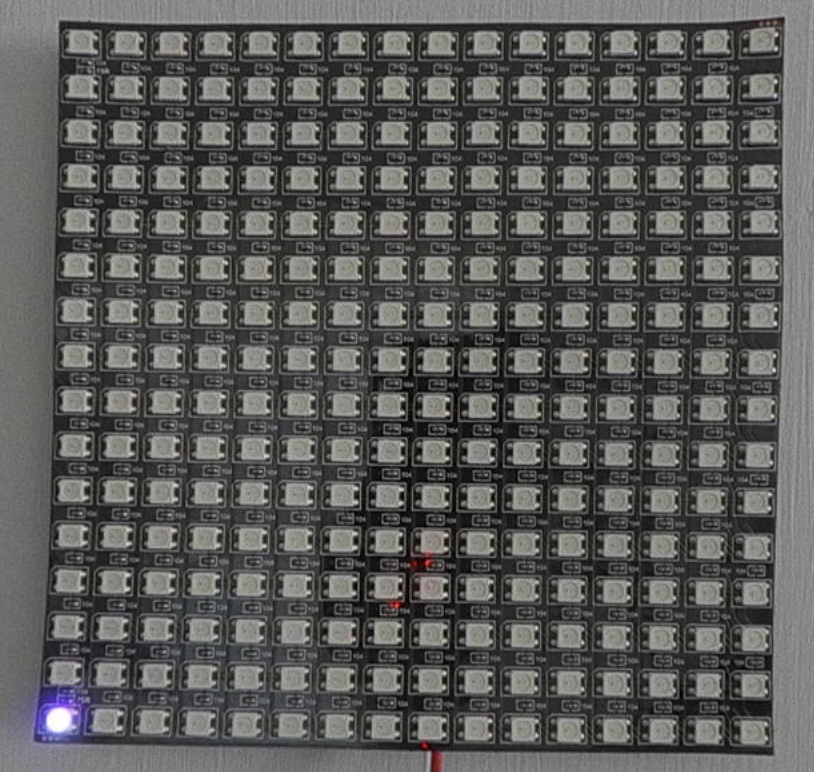
Now let’s try to put equal numbers of a lower intensity and see happens
from wc import *
canvas = Canvas()
white = (20,20,20)
canvas.add(Point(0,0,white))
As you can see, the color is still white but the intensity is diminished.
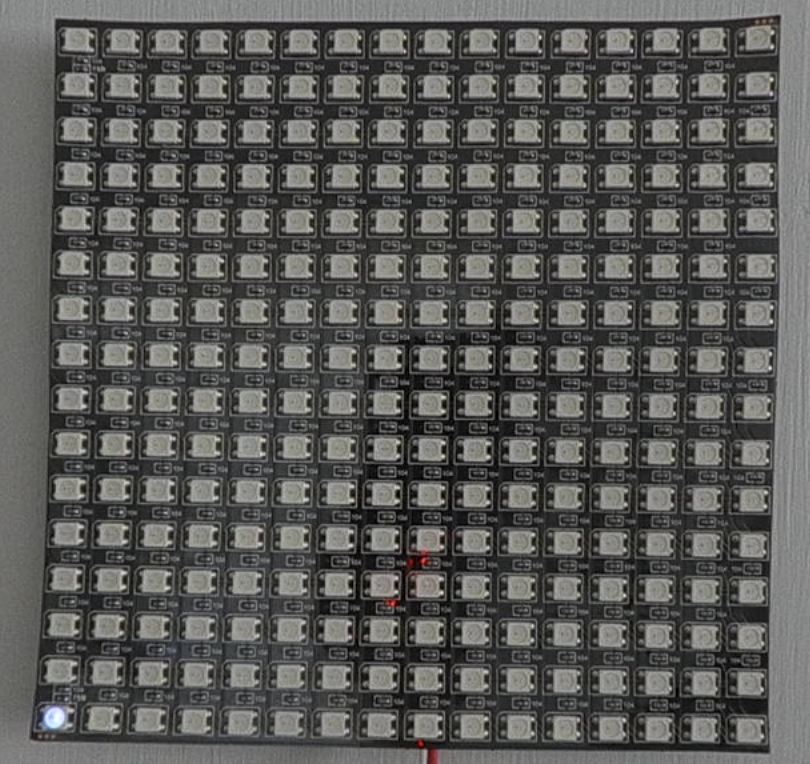
An alternate way to change the intensity of an LED is to pass a fourth parameter to the Point
constructor. This parameter can vary from 0 to 100 where 0 is effectively an off statement and 100 is the maximum brightness the LED can produce. If you execute the code below you will see that the brightness dramatically increases. This is because the default brightness if no parameter is passed in is 1.
from wc import *
canvas = Canvas()
white = (255,255,255)
canvas.add(Point(0,0,white,100))
Color Combinations
Some colors look good together and some colors don’t look so great together. You’ve probably seen examples of this when looking at combinations of clothes that you can wear or the colors you can use to paint the walls in your house. There is actually a theory behind pleasing color palettes. We won’t get into this very deeply except to use an online tool that helps visualize colors. Click on the link below to try it out.
https://color.adobe.com/create/color-wheel
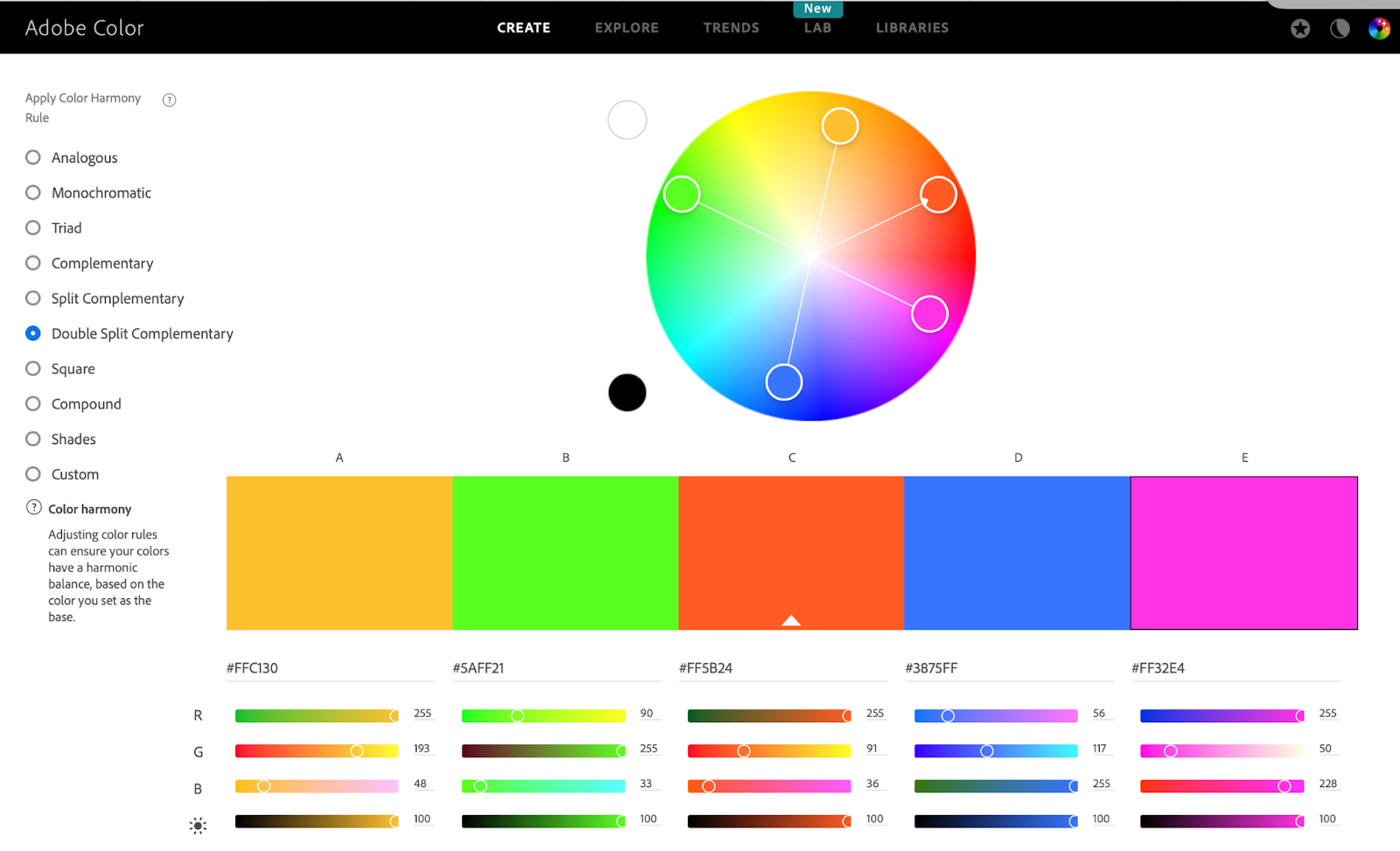
This application lists the individual intensities for each color on the bottom of the page and a hexadecimal value at the top. For example FFC130
produces an orange color. You can copy these values into your code. For the colors above, your code would like something like this.
from wc import *
canvas = Canvas()
canvas.add(
[Point(0,0,'FFC130'),
Point(1,0,'5AFF21'),
Point(2,0,'FF5B24'),
Point(3,0,'3875FF'),
Point(4,0,'FF32E4')
])
Discussion
Try using the color wheel application to generate different colors. See how closely the colors match the color wheel.
Try coding different intensities of red by varying the value from 0 to 255. Is there a value at which the LED no longer turns on?
What happens if we type the wrong color name in our program? Try the following and look at the output window.
from wc import *
canvas = Canvas()
canvas.add(Point(0,0,reddish))
In the output window you will see the following message.
code.py output:
Traceback (most recent call last):
File "code.py", line 4, in <module>
NameError: name 'reddish' is not defined
As you can see, the output window is very useful! Not only did it tell you that the term reddish has not been defined, it even tells you the line number where to look! The Thonny editor displays the line numbers conveniently for you as shown below so finding line 4 is very easy.
Summary
In this lesson we discussed how an LED light strip is able to make many different colors by mixing different intensities of red, green, and blue. We will use this feature in our designs to make a whole library of visually exciting patterns.
No responses yet