In this post we are going to discuss how to add some randomizing features in to your code.
Introduction
There are many cases when we want our code to always do the same things every time. For example, a stop light should always cycle the lights in a predictable manner where it cycles from green, to yellow, to red and then back to green. We also want our microwave oven to really adhere to the cooking time that we enter in the timer. But there are cases where we want our system to behave in an unpredictable manner. For example, in game design we don’t want the challenges that we present to the player to be too predictable, otherwise they could just memorize the sequence of events and always win. In computer programming we rely on randomizers to introduce that uncertainty that we need in our programs.
Randomizer Functions
In order to use randomizer functions we need to import a library. This is done using the following statement at the top of our program.
import random
There are two randomizer functions we will discuss in this post. The first function randrange(min, max)
returns a value within a range that is expressed via a minimum and maximum parameter. Let’s use this function to create lines of alternating color with random length.
from wc import *
import random
canvas = Canvas()
color1 = (255, 193, 48)
color2 = (56, 117, 255)
color = color1
lines = []
for y in range(canvas.height):
pos = 0
while pos < canvas.width:
length = random.randrange(1, 10)
if (pos + length >= canvas.width):
length = canvas.width - pos - 1
if color == color1:
color = color2
else:
color = color1
lines.append(HLine(pos, y, length, color, 1))
pos += length + 1
canvas.add(lines)
Running this code will produce a pattern that looks similar to this. Your pattern will not be identical because each time you run this program you will get a different result.
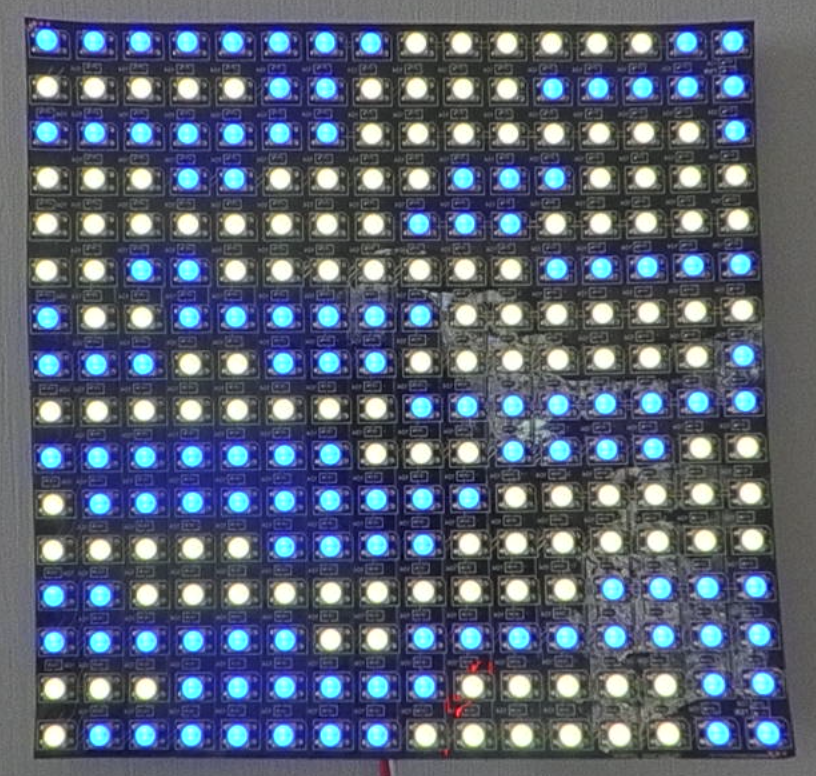
Let’s put this code in a loop so that we can see how each iteration produces a new result. Try running the code below and see what happens.
from wc import *
import random
import time
canvas = Canvas()
color1 = (255, 193, 48)
color2 = (56, 117, 255)
color = color1
while True:
lines = []
for y in range(canvas.height):
pos = 0
while pos < canvas.width:
length = random.randrange(1, 10)
if (pos + length >= canvas.width):
length = canvas.width - pos - 1
if color == color1:
color = color2
else:
color = color1
lines.append(HLine(pos, y, length, color, 1))
pos += length + 1
canvas.remove_all(update=False)
canvas.add(lines)
time.sleep(.1)
Running this program produces the result shown below.
Let’s now take a look at the second randomizer function called random.choice()
. This function allows you to randomly pick from a set of values. In the code below we define five colors and the choice function will select one of the values from the set.
from wc import *
import random
import time
canvas = Canvas()
color1 = (255, 193, 48)
color2 = (56, 117, 255)
color3 = (255, 91, 36)
color4 = (90, 255, 33)
color5 = (255, 50, 228)
while True:
lines = []
for y in range(canvas.max_y):
pos = 0
while pos < canvas.max_x:
length = random.randrange(1, 10)
if (pos + length >= canvas.max_x):
length = canvas.max_x - pos - 1
color = random.choice([color1, color2, color3, color4, color5])
lines.append(HLine(pos, y, length, color, 1))
pos += length + 1
canvas.remove_all(update=False)
canvas.add(lines)
time.sleep(.1)
Running this code will produce a series of color patterns such as the one shown below.
Summary
In this post we learned how to use two randomizer functions to produce some fun patterns on our matrix. You can use this concept in your own projects when an exact result is either not needed or not desirable. This needs arises often when creating artwork and games.
No responses yet